Java pattern program enhances the coding skill, logic, and looping concepts. It is mostly asked in Java interview to check the logic and thinking of the programmer. We can print a Java pattern program in different designs. To learn the pattern program, we must have a deep knowledge of the Java loop, such as for loop do-while loop. In this section, we will learn how to print a pattern in Java.
We have classified the Java pattern program into three categories:
Start Pattern
Number Pattern
Character Pattern
Before moving to the pattern programs, let's see the approach.
Whenever you design logic for a pattern program, first draw that pattern in the blocks, as we have shown in the following image. The figure presents a clear look of the pattern.
Each pattern program has two or more than two loops. The number of the loop depends on the complexity of pattern or logic. The first for loop works for the row and the second loop works for the column. In the pattern programs, Java for loop is widely used.
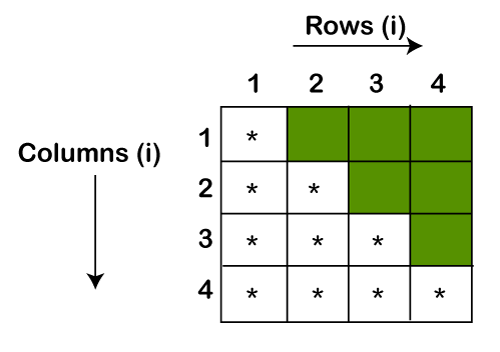
In the above pattern, the row is denoted by i and the column is denoted by j. We see that the first row prints only a star. The second-row prints two stars, and so on. The colored blocks print the spaces.
Let's create the logic for the pattern, give above. In the following code snippet, we are starting row and column value from 0. We can also start it from 1, it's your choice.
- for(int i=0; i<row; i++)
- {
- for(int j=0; j<=i; j++)
- {
- System.out.print("* ");
- }
- System.out.println();
In the above code snippet, the first for loop is for row and the second for loop for columns.
Let's see the execution of the code step by step, for n=4 (the number of rows we want to print).
Iteration 1:
For i=0, 0<4 (true)
For j=0, j<=0 (true)
The first print statement prints a star at the first row and the second println statement prints the spaces and throws the cursor at the next line.
- *
Now the value of i and j is increased to 1.
Iteration 2:
For i=1, 1<4 (true)
For j=1, 1<=1 (true)
The first print statement prints two stars at the second row and the second println statement prints the spaces and throws the cursor at the next line.
- *
- * *
Now the value of i and j is increased to 2.
Iteration 3:
For i=2, 2<4 (true)
For j=2, 2<=2 (true)
The first print statement prints three stars at the third row and the second println statement prints the spaces and throws the cursor at the next line.
- *
- * *
- * * *
Now the value of i and j is increased to 3.
Iteration 4:
For i=3, 3<4 (true)
For j=3, 3<=3 (true)
The first print statement prints four stars at the fourth row and the second println statement prints the spaces and throws the cursor at the next line.
- *
- * *
- * * *
- * * * *
Now the value of i and j is increased to 4.
For i=4, 4<4 (false)
The execution of the program will terminate when the value of i will be equal to the number of rows.
Star Pattern
1. Right Triangle Star Pattern
- public class RightTrianglePattern
- {
- public static void main(String args[])
- {
- //i for rows and j for columns
- //row denotes the number of rows you want to print
- int i, j, row=6;
- //outer loop for rows
- for(i=0; i<row; i++)
- {
- //inner loop for columns
- for(j=</sp