The object-oriented programming approach allows a complex code snippet to divide into smaller code blocks by creating objects. These objects mutually share two characteristics: state and behavior.
Here are some of the OOPs elements that we are going to discuss with Kotlin code examples:
- Class and Objects
- Constructors
- Inheritance
- Abstract Class

Class in Kotlin
The first before creating an object, we need to define a class that is also known as the blueprint for the object.
Syntax:
class ClassName {
// property
// member function
... .. ...
}
Objects in Kotlin
While defining a class, we only define the specifications for the object, no other parameter like memory or storage is allocated.
Syntax:
var obj1 = ClassName()
Constructors in Kotlin
A constructor is a way to initialize class properties. It’s a member function called when an object is instantiated. But in Kotlin, it works differently.
There are two types of constructors in Kotlin:
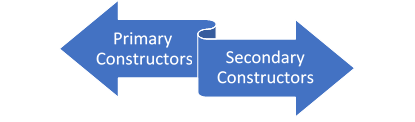
Constructors in Kotlin
Primary constructor: Optimized way to initialize a class
Syntax:
class myClass(valname: String,varid: Int) {
// class body
}
Secondary constructor: Helps to add initialization logic
Kotlin Inheritance
Inheritance occurs when some properties of the parent class are acquired by the child class. Inheritance is allowed when two or more classes have the same properties.
Syntax:
open class ParentClass(primary_construct){
// common code
}class ChildClass(primary_construct): ParentClass(primary_construct_initializ){
// ChildClass specific behaviours
}
Abstract Class in Kotlin
An abstract class is a class that cannot be instantiated, but we can inherit subclasses from them. ‘abstract ‘ keyword is used to declare an abstract class.
For Example 6:
open class humanbeings {
open fun Eat() {
println("All Human being Eat")
}
}
abstract class Animal : humanbeings() {
override abstract fun Eat()
}
class Cat: Animal(){
override fun Eat() {
println("Cats also loves eating")
}
}
fun main(args: Array<String>){
val lt = humanbeings()
lt.Eat()
val d = Cat()
d.Eat()
}
Output: